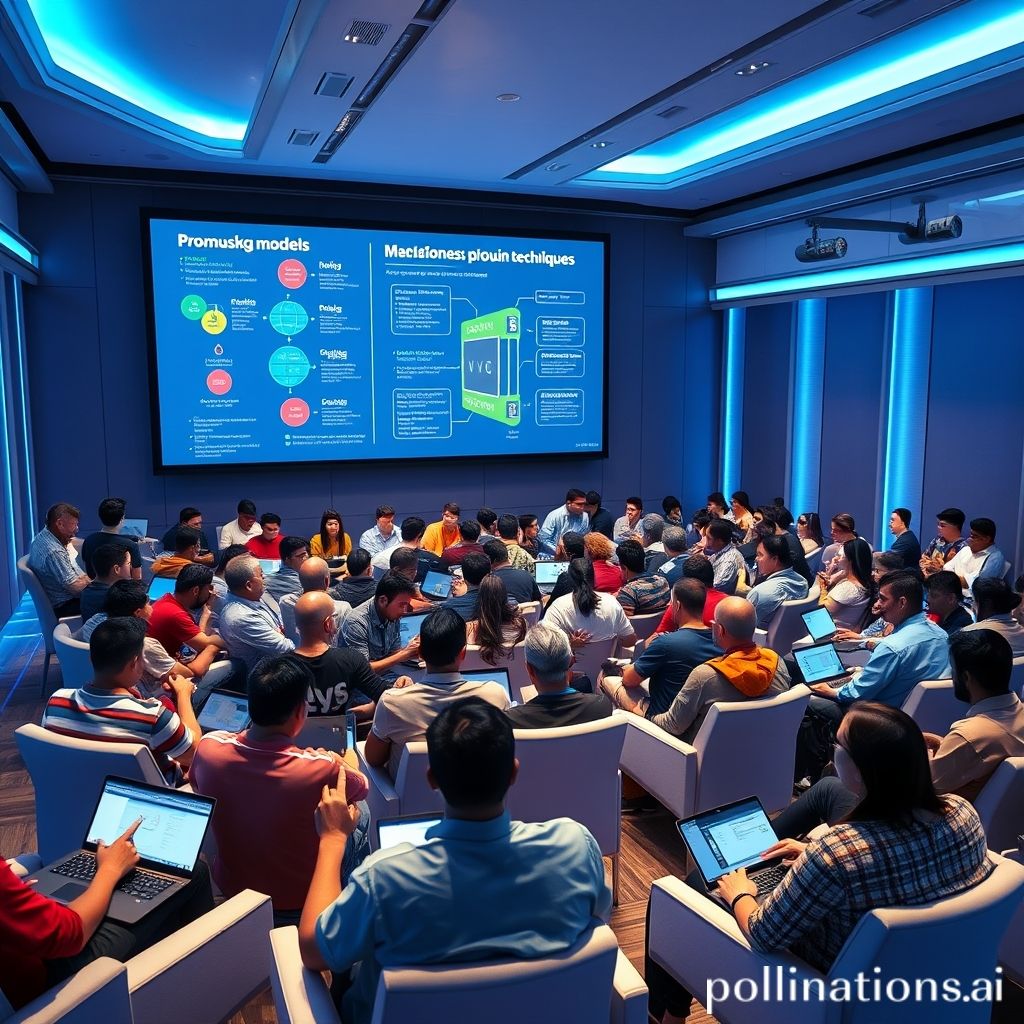
Asynchronous Programming Techniques
Asynchronous programming is a programming paradigm that allows for non-blocking operations, enabling developers to write more efficient code. It is particularly useful in situations where tasks may take time to complete, such as network calls, file I/O operations, or database queries. By leveraging asynchronous techniques, programmers can ensure that their applications remain responsive and can handle multiple operations concurrently.
Understanding Asynchronous Programming
In traditional synchronous programming, operations are executed sequentially, blocking the execution thread until each operation completes. This can lead to performance bottlenecks, especially in applications with heavy I/O operations. Asynchronous programming, on the other hand, allows multiple operations to be initiated before previous operations have completed. This is achieved through callbacks, promises, and async/await constructs that facilitate a more flexible approach to managing control flow.
Callbacks
Callbacks are functions that are passed as arguments to other functions and are executed after the completion of a task. They are one of the earliest forms of asynchronous programming. While callbacks allow for non-blocking behavior, they can lead to "callback hell," where the code becomes difficult to read and maintain due to deeply nested functions. This technique requires careful management of error handling and execution order.
Promises
Promises offer a more structured way to handle asynchronous operations compared to callbacks. A promise is an object that represents the eventual completion (or failure) of an asynchronous operation and its resulting value. Promises can be in one of three states: pending, fulfilled, or rejected. The `then()` and `catch()` methods can be used to handle the resolved value or the error, promoting cleaner and more manageable code. Promises also support chaining, allowing multiple asynchronous operations to be executed in sequence.
Async/Await
Introduced in ECMAScript 2017, the async/await syntax provides a way to write asynchronous code that looks and behaves like synchronous code. The `async` keyword is used to declare a function as asynchronous, and within this function, the `await` keyword can be used to pause the execution until the promise is resolved. This approach makes it easier to read and understand asynchronous code, reduces the complexity of handling multiple promises, and prevents callback hell.
Event Loop
The event loop is a fundamental part of asynchronous programming in JavaScript. It is responsible for executing code, collecting and processing events, and executing queued sub-tasks. The event loop enables the execution of asynchronous callbacks and periodically checks for pending tasks, ensuring that the system remains responsive. Understanding the event loop is crucial for developers working with asynchronous techniques, as it underpins how and when tasks are executed.
Conclusion
Asynchronous programming techniques are essential for building efficient, high-performance applications. By utilizing approaches such as callbacks, promises, and the async/await syntax, developers can write code that is not only non-blocking but also easier to read and maintain. With a solid understanding of these techniques and the event loop, developers can effectively manage asynchronous operations and enhance the user experience in their applications.