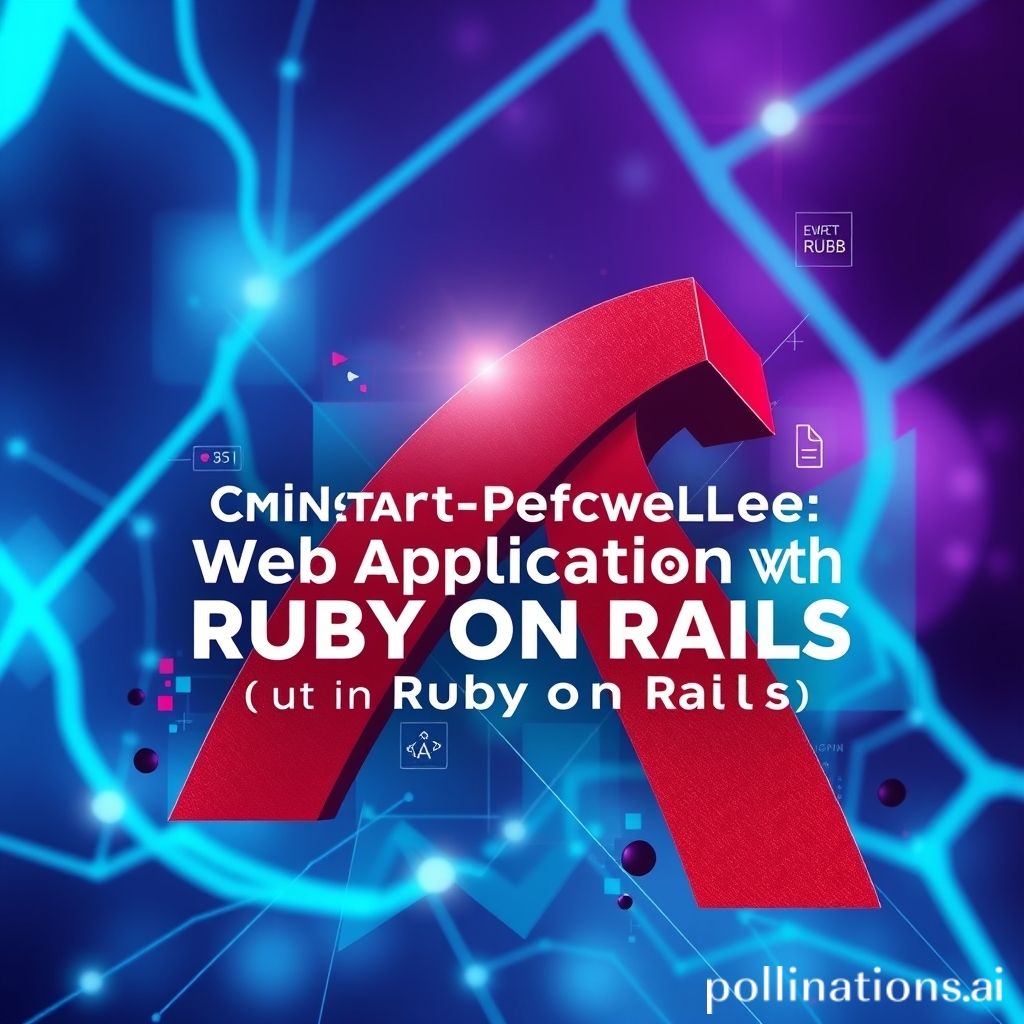
Introduction
Ruby on Rails (RoR), often simply referred to as Rails, is a powerful web application framework that has gained immense popularity for its ability to facilitate rapid development of high-performance web applications. This article will outline the key features and best practices for building high-performance web applications using Ruby on Rails.
Why Choose Ruby on Rails?
Ruby on Rails is favored for several reasons:
- Convention over Configuration: Rails follows the principle of convention over configuration, which means developers can focus on writing code without needing to worry about setting up configurations.
- RESTful Architecture: Rails makes it easy to develop applications that adhere to the RESTful architectural style, promoting better organization of web services.
- Community and Gems: The Rails community is vast, providing a wealth of libraries (gems) that can be utilized to enhance application functionality quickly.
Best Practices for High-Performance Web Applications
To build high-performance web applications with Rails, consider the following best practices:
1. Optimize Database Queries
Database queries can significantly impact application performance. To optimize:
- Use
includes
andeager_load
to reduce N+1 query problems. - Index frequently queried columns to speed up lookups.
- Utilize database pagination to manage large datasets efficiently.
2. Implement Caching Strategies
Caching can drastically improve response times. Consider using:
- Fragment caching for partial views.
- Action caching for controller actions.
- Page caching for static pages that do not change frequently.
3. Optimize Asset Pipeline
Rails comes with an asset pipeline, but optimizing it is essential for performance:
- Minimize CSS and JavaScript using built-in tools.
- Use CDNs (Content Delivery Networks) to deliver assets faster to users globally.
- Leverage gzip compression for assets to reduce transmission time.
4. Use Background Jobs
Heavy tasks such as sending emails or processing images can slow down user experience. Offload these tasks using background job processors like:
- Sidekiq
- Resque
- Delayed Job
Testing and Monitoring Performance
To maintain high performance, testing and monitoring are crucial:
- Use tools like Rails' built-in testing framework for unit tests and integration tests.
- Monitor application performance with tools like New Relic or Skylight to identify bottlenecks.
- Benchmark critical parts of your application to ensure they meet performance expectations.
Conclusion
Building high-performance web applications with Ruby on Rails requires following best practices for optimization and leveraging the framework's powerful features. By focusing on efficient database queries, implementing effective caching strategies, optimizing the asset pipeline, and using background jobs, developers can create robust applications that meet user needs and scale effectively.