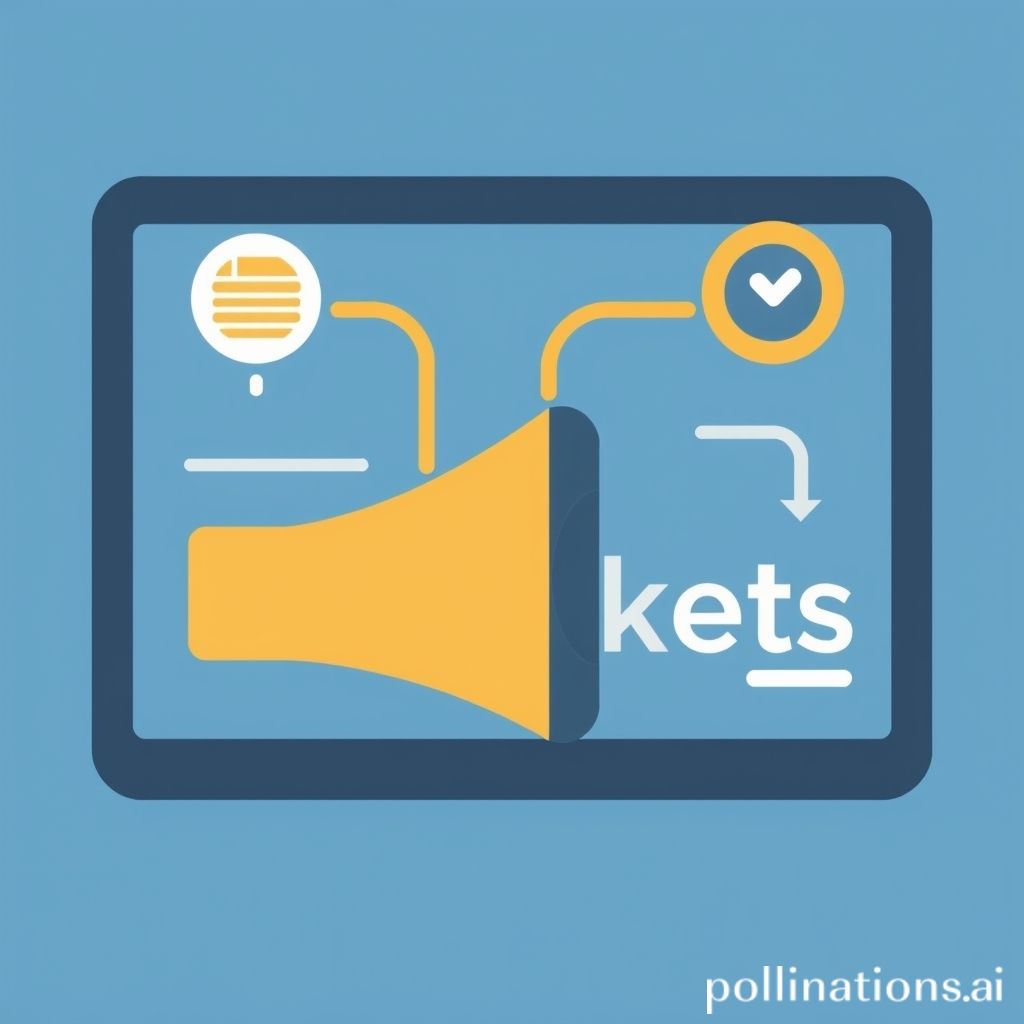
Building Real-Time Applications with WebSockets
In today's fast-paced digital landscape, the demand for real-time applications is growing exponentially. These applications provide instantaneous updates and seamless interactions, making them essential for online gaming, collaboration tools, live notifications, and chat applications. One of the most effective technologies for building real-time applications is WebSockets, a protocol that allows for a persistent connection between a client and a server.
WebSockets provide a full-duplex communication channel over a single TCP connection, enabling both the client and server to send messages independently without the overhead of frequent HTTP requests. This efficiency reduces latency and improves performance, making WebSockets an ideal choice for real-time features.
Understanding WebSockets
The WebSocket protocol was standardized in 2011 and is designed to work seamlessly alongside HTTP. Unlike traditional HTTP requests, which require a client to initiate communication, WebSockets maintain an open connection once established, allowing for continuous data exchange. To initiate a WebSocket connection, the client starts an HTTP handshake that upgrades the connection to a WebSocket connection if both parties support it.
This upgrade is crucial because it allows for messages to be sent in both directions simultaneously, significantly enhancing the user experience. Once the connection is established, messages can be sent as text or binary data, making WebSockets versatile for various applications.
Setting Up a WebSocket Server
To create a real-time application using WebSockets, you first need to set up a WebSocket server. Various programming languages and frameworks offer built-in support for WebSockets. For example, Node.js, utilizing the ws library, makes it simple to create a WebSocket server. Here’s a basic outline:
const WebSocket = require('ws'); const server = new WebSocket.Server({ port: 8080 }); server.on('connection', (socket) => { socket.on('message', (message) => { console.log('Received:', message); socket.send(`Echo: ${message}`); }); });
In this example, whenever a client connects to the server, it can send messages that the server echoes back, demonstrating the two-way communication WebSockets provide.
Client-Side Implementation
On the client side, you can easily create a WebSocket connection using JavaScript. Here’s a simple implementation:
const socket = new WebSocket('ws://localhost:8080'); socket.onopen = () => { console.log('Connected to the server'); socket.send('Hello Server!'); }; socket.onmessage = (event) => { console.log('Message from server:', event.data); };
This code connects to the server and sends a message once the connection is established. It also listens for incoming messages and logs them to the console.
Use Cases for WebSockets
WebSockets are ideal for a range of applications requiring live data updates. Some common use cases include:
- Real-time chat applications
- Collaborative platforms (like Google Docs)
- Online gaming
- Financial trading applications
- Live sports updates
Conclusion
Utilizing WebSockets can dramatically enhance the user experience of your applications, providing real-time data updates and interactions. As user expectations shift towards instant communication, incorporating WebSocket technology can set your application apart in a competitive landscape.