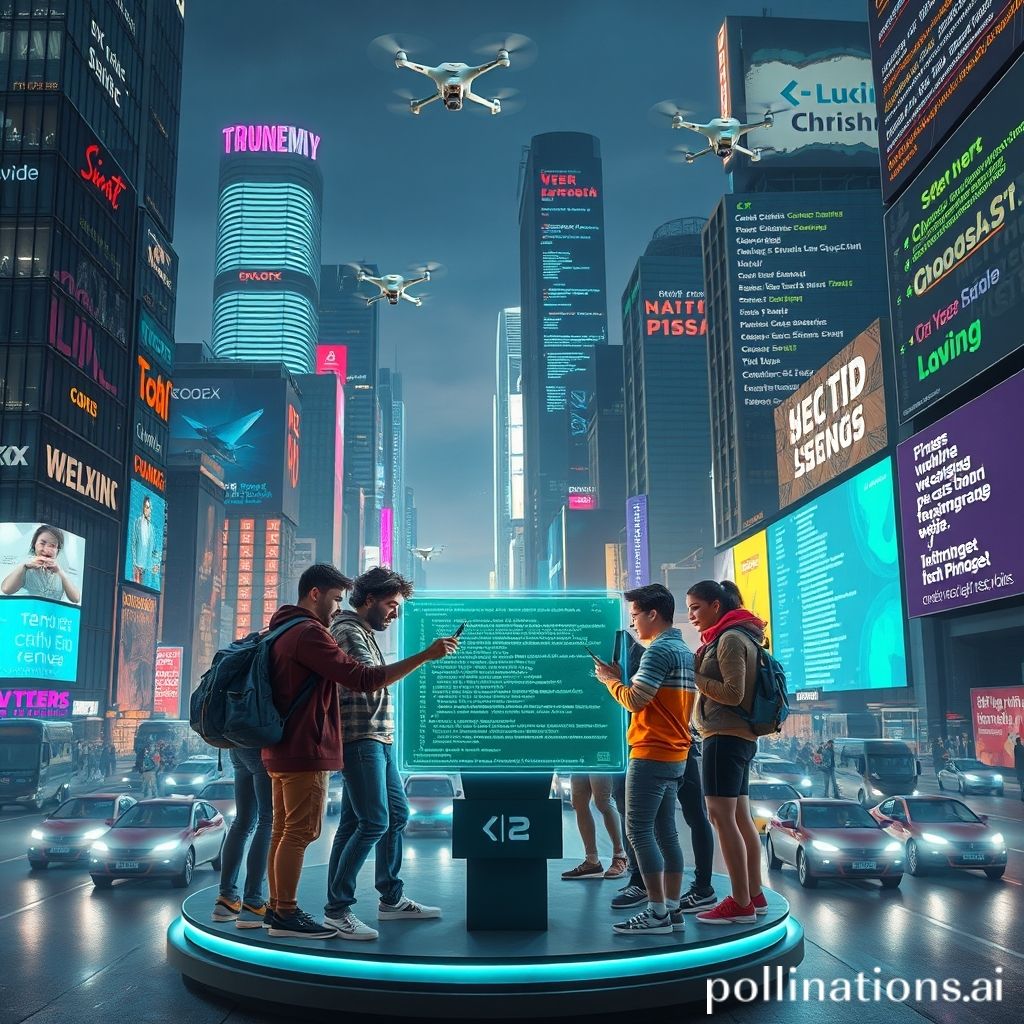
Building Robust APIs with Flask
Flask is a micro web framework for Python that is widely used for developing web applications and RESTful APIs. Its simplicity and flexibility make it a preferred choice for developers who want to create robust and efficient APIs. In this article, we will explore how to build a robust API with Flask, covering key concepts and best practices.
Setting Up Flask
To get started with Flask, you'll need to install the framework. You can do this using pip:
pip install Flask
Once installed, you can create a simple Flask application with just a few lines of code:
from flask import Flask, jsonify app = Flask(__name__) @app.route('/api', methods=['GET']) def home(): return jsonify({"message": "Welcome to the Flask API!"}) if __name__ == '__main__': app.run(debug=True)
Creating Endpoints
Endpoints are crucial parts of any API. They define the routes that clients will use to access resources. In Flask, you can easily create multiple endpoints:
@app.route('/api/items', methods=['GET']) def get_items(): items = [{"id": 1, "name": "Item 1"}, {"id": 2, "name": "Item 2"}] return jsonify(items) @app.route('/api/items', methods=['POST']) def create_item(): new_item = request.get_json() return jsonify(new_item), 201
Handling Requests and Responses
Understanding how to handle requests and return responses is essential in API development. Flask provides tools to manage request data and customize responses easily:
- Use
request
to access incoming request data. - Utilize
jsonify
for formatting responses as JSON. - Handle different HTTP methods (GET, POST, PUT, DELETE) effectively.
Validation and Error Handling
Robust APIs require proper validation and error handling. You can implement validation using libraries like Marshmallow
:
- Define schemas to validate input data.
- Return appropriate error messages on validation failure.
from marshmallow import Schema, fields, ValidationError class ItemSchema(Schema): id = fields.Int(required=True) name = fields.Str(required=True) @app.route('/api/items', methods=['POST']) def create_item(): try: item_data = ItemSchema().load(request.get_json()) return jsonify(item_data), 201 except ValidationError as err: return jsonify(err.messages), 400
Authentication and Authorization
To secure your API, consider implementing authentication and authorization mechanisms. Popular options include:
- Token-based authentication with JWT (JSON Web Tokens).
- OAuth2 for third-party access.
Conclusion
Building robust APIs with Flask can be a straightforward process once you understand the framework's core concepts. By following best practices like data validation, error handling, and implementing authentication, you can create powerful APIs that serve your application’s needs. Whether for simple projects or more extensive applications, Flask offers the versatility required to build reliable APIs efficiently.