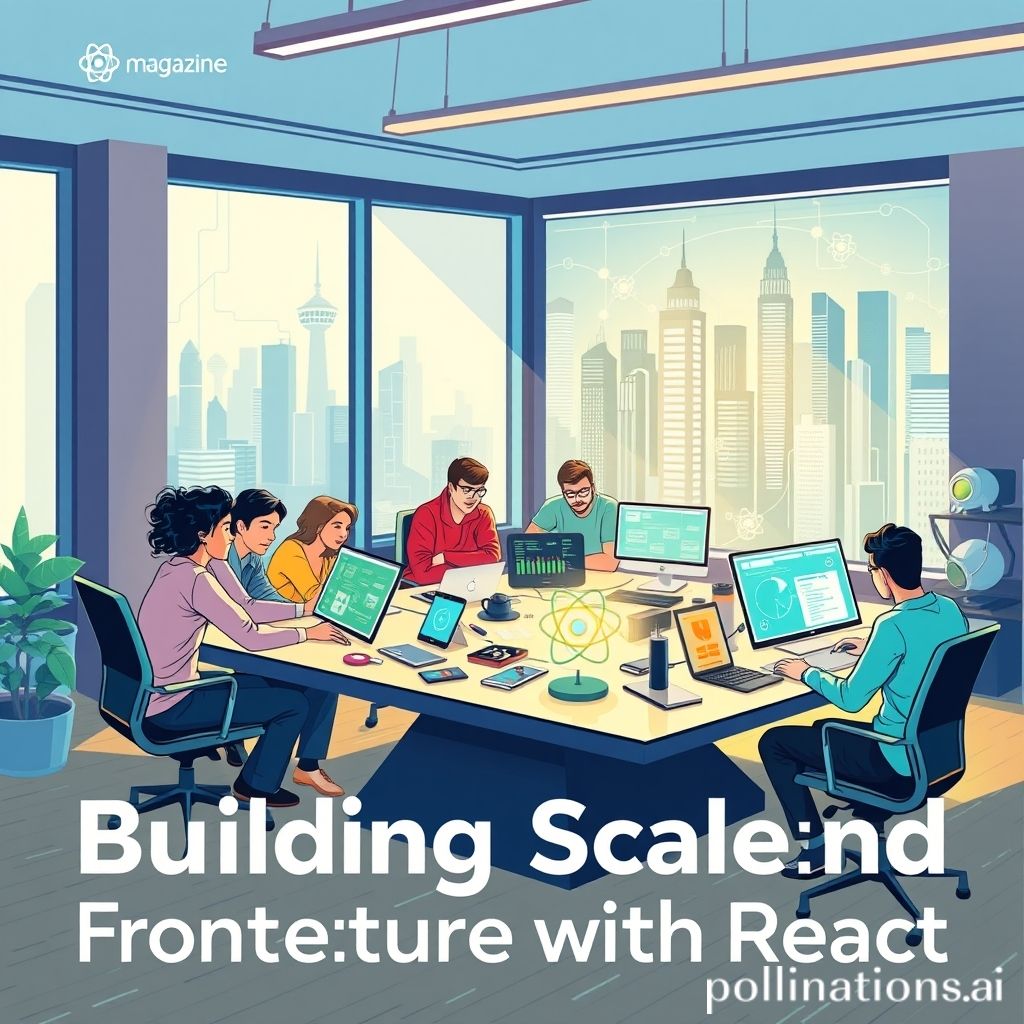
Building Scalable Frontend Architectures with React
In the fast-evolving world of web development, building scalable frontend architectures is crucial for maintaining an efficient and manageable codebase. With the rise of JavaScript frameworks, React has emerged as a leading choice for developers seeking to create dynamic and responsive user interfaces. This article outlines essential strategies for building scalable architectures with React.
1. Component-Based Architecture
React is centered around a component-based architecture, which promotes reusability and separation of concerns. Components can be thought of as reusable building blocks that encapsulate specific functionality.
- Reusable Components: Create small, focused components that can be reused across different parts of the application.
- Container and Presentational Components: Separate logic and UI into container components that handle state and presentational components that focus on rendering UI.
- Higher-Order Components: Use higher-order components (HOCs) for cross-cutting concerns, enhancing functionality without modifying existing components.
2. State Management
As applications grow in size and complexity, managing state becomes a critical challenge. Efficient state management ensures that your application remains responsive and organized.
- Local State: Utilize React's built-in state management for local component states.
- Context API: Leverage the Context API for sharing global data without prop drilling.
- External State Management Libraries: Consider using libraries like Redux or MobX for more complex state management needs.
3. Code Splitting
Code splitting allows you to load only the necessary code for the current view, improving initial load times and overall performance.
- Dynamic Imports: Use dynamic imports to load components only when they are needed, rather than at the initial load.
- React.lazy and Suspense: Implement React.lazy and Suspense for easily handling lazy-loaded components, ensuring a seamless user experience.
4. Styling Strategies
Choosing the right styling approach is essential for maintaining a scalable architecture. Different strategies suit different project needs.
- CSS Modules: Use CSS Modules for scoped styling, avoiding conflicts in larger applications.
- Styled Components: Consider styled-components for styling components using a CSS-in-JS approach.
- Utility-First Frameworks: Explore utility-first CSS frameworks like Tailwind CSS for rapidly building custom designs.
5. Testing and Quality Assurance
Building scalable applications necessitates a strong testing strategy to ensure maintainability and performance over time.
- Unit Testing: Utilize tools like Jest and Enzyme to conduct unit tests on individual components.
- Integration Testing: Implement integration tests to verify that components interact correctly with each other.
- End-to-End Testing: Employ end-to-end testing frameworks like Cypress for testing entire application workflows.
Conclusion
Building scalable frontend architectures with React requires careful planning and execution. By embracing a modular component-based approach, efficient state management, code splitting, appropriate styling strategies, and robust testing, developers can create applications that not only perform well but are also easy to maintain and extend as user needs evolve.