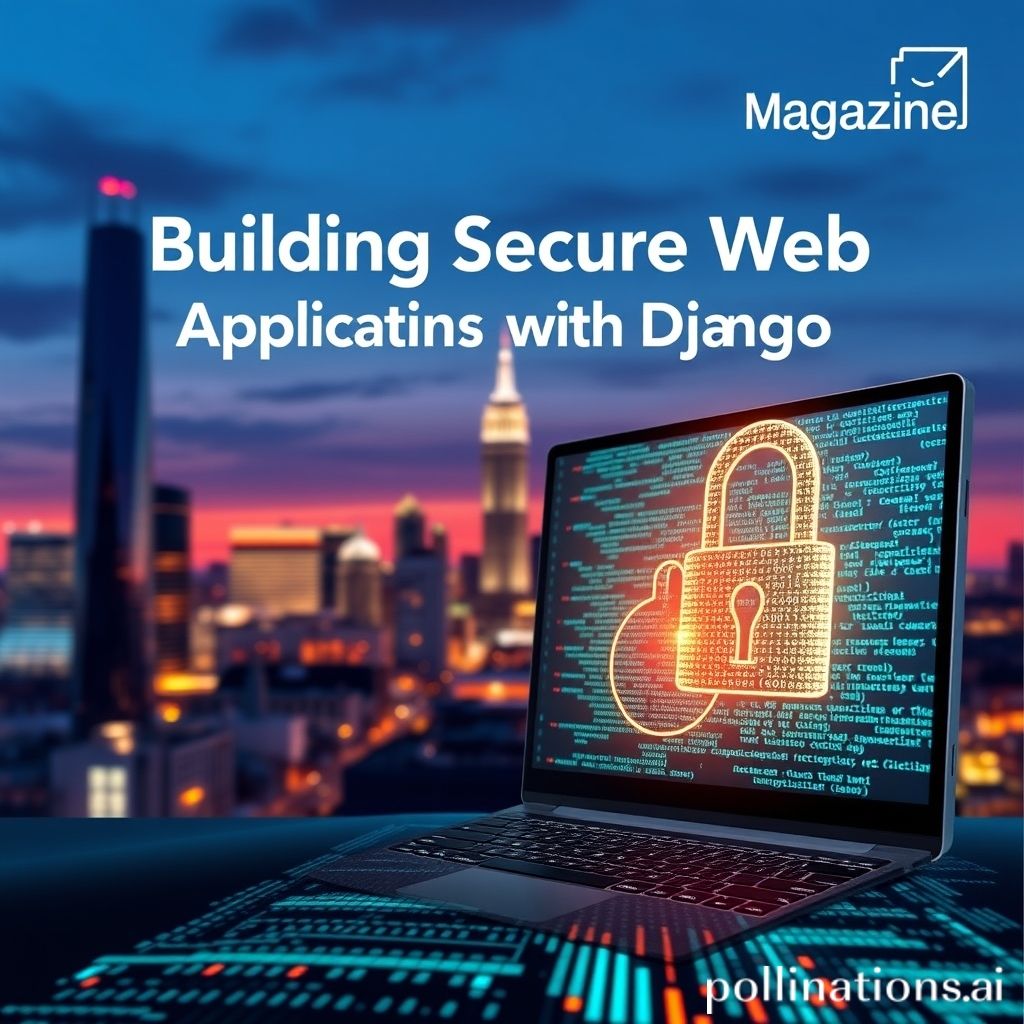
Building Secure Web Applications with Django
In today's digital landscape, security is paramount when developing web applications. Django, a high-level Python web framework, is designed with security in mind, offering built-in features to help developers create robust applications. This article discusses key practices for building secure web applications with Django.
1. Use the Latest Version of Django
Always ensure that you are using the latest stable version of Django. The framework regularly receives updates that include security patches and improvements. By using the most recent version, you take advantage of these enhancements and minimize the risk of vulnerabilities.
2. Implement Proper Authentication
Authentication is the first line of defense for any application. Django provides a powerful authentication system, which includes:
- Built-in user management.
- Password hashing using modern algorithms.
- Support for user sessions and permissions.
Always use Django’s built-in tools to manage authentication rather than creating your own system. This approach minimizes security risks.
3. Use HTTPS
Using HTTPS is crucial for protecting data transmitted between the client and the server. Ensure you have an SSL/TLS certificate installed and configure your Django application to redirect all HTTP traffic to HTTPS. You can use the following setting in your settings.py file:
SECURE_SSL_REDIRECT = True
4. Protect Against CSRF Attacks
Django has built-in protection against Cross-Site Request Forgery (CSRF) attacks. Always use the CSRF middleware, which is enabled by default in Django. Include the CSRF token in forms to ensure that requests are coming from authenticated sessions. For example:
- Use
{% csrf_token %}
in your HTML forms.
5. Sanitize User Input
Malicious users can exploit vulnerabilities through unsanitized input. Django provides mechanisms to escape HTML and validate form data. Use Django forms and model validation to ensure data integrity:
- Utilize
clean()
methods in Django forms to validate and sanitize user input. - Escape output with the
safe
filter only when necessary.
6. Utilize Django’s Security Features
Django includes various security features that help safeguard your application:
SECURE_BROWSER_XSS_FILTER = True
to enable the browser's XSS filtering.SECURE_CONTENT_TYPE_NOSNIFF = True
to prevent browsers from MIME-sniffing.SECURE_HSTS_SECONDS
to enable HTTP Strict Transport Security (HSTS).
7. Regularly Audit and Test Security
Conduct regular security audits and code reviews to identify vulnerabilities. Utilize tools like Bandit
or Django’s security check
commands. Penetration testing is also recommended to uncover potential weaknesses in your application.
Conclusion
Building secure web applications with Django involves utilizing its built-in features and following best practices for security. By staying up-to-date with security updates, implementing proper authentication, and regularly testing your application, you can create a more secure web environment for your users.