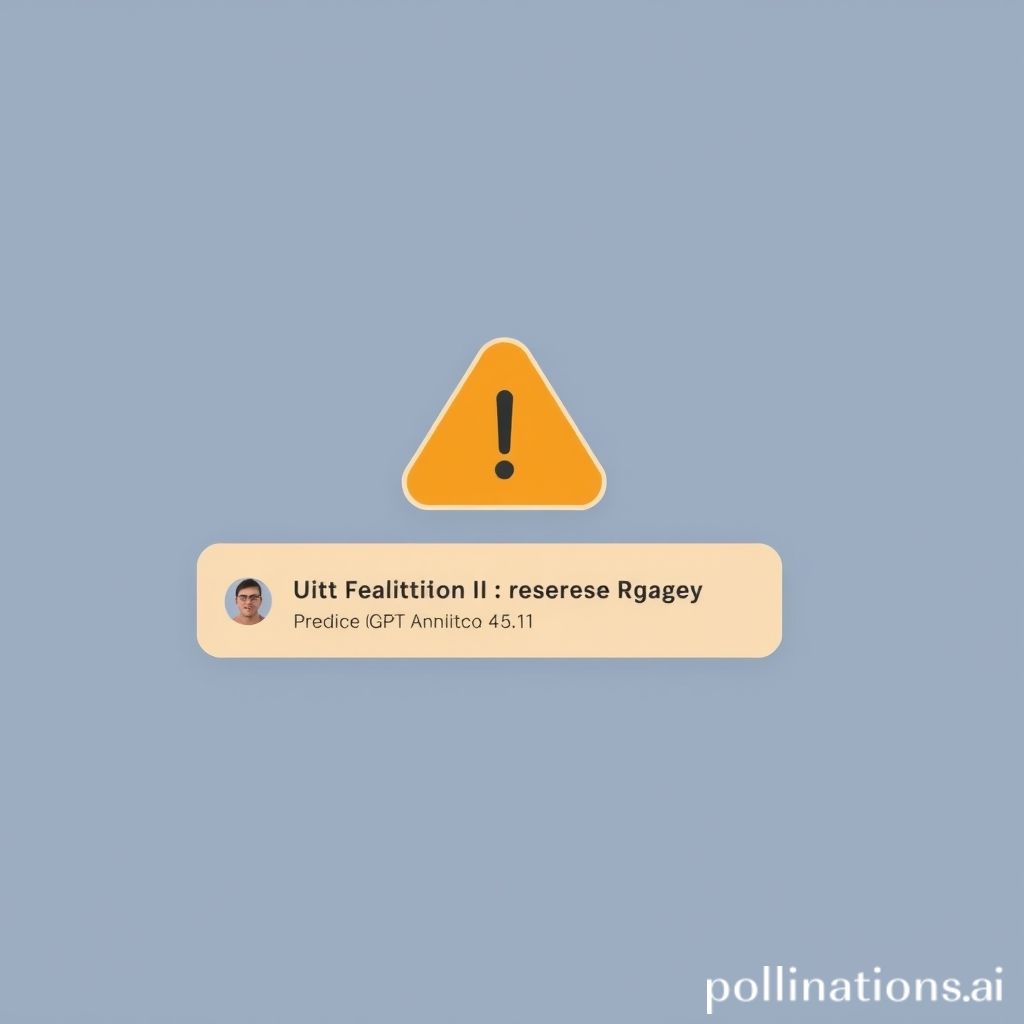
Creating Dynamic Web Applications with Angular
Angular is a powerful, open-source web application framework developed by Google, designed to build robust and scalable dynamic web applications. With its component-based architecture, Angular allows developers to create reusable UI components, making it a popular choice among developers for creating single-page applications (SPAs).
Getting Started with Angular
To begin developing with Angular, you need to set up your environment. The easiest way to start is by using the Angular CLI (Command Line Interface), which streamlines the process of creating and managing Angular applications.
- Install Node.js and npm (Node Package Manager).
- Run the command
npm install -g @angular/cli
to install the Angular CLI globally. - Create a new Angular project using
ng new your-project-name
. - Navigate to your project folder with
cd your-project-name
. - Run
ng serve
to start the development server.
Understanding the Angular Architecture
Angular's architecture is built around components, modules, and services. Each component is a self-contained piece of the UI, encapsulating its own data and behavior. Modules help organize application components into cohesive blocks, while services are used for sharing data and logic across components.
- Components: Define the views, encapsulating HTML, CSS, and logic.
- Modules: Group related components, services, and other functionalities, enabling lazy loading and better organization.
- Services: Provide reusable methods and facilitate data sharing across components.
Data Binding and Directives
One of Angular's core features is its powerful data binding. There are two main types of data binding in Angular: one-way data binding and two-way data binding.
- One-Way Data Binding: Allows data to flow in one direction, either from the component to the view or vice versa.
- Two-Way Data Binding: Facilitates synchronization between the view and the model, ensuring that any changes in the view reflect in the model and vice versa.
Additionally, Angular provides directives, which are special markers in the DOM that allow developers to extend HTML with custom behavior.
Building a Simple To-Do Application
To illustrate how to create a dynamic web application with Angular, let’s build a simple To-Do application. This app allows users to add, delete, and mark tasks as completed.
- Create a new component for the To-Do list using
ng generate component todo
. - Set up a service to manage the to-do items, facilitating data storage.
- Use Angular Forms to capture user input for new tasks.
- Implement methods to handle adding, deleting, and displaying tasks within the component.
Conclusion
Create dynamic and interactive web applications using Angular is a straightforward process thanks to its robust features and component-based architecture. With Angular, developers can build applications that are not only functional but also maintainable and scalable.