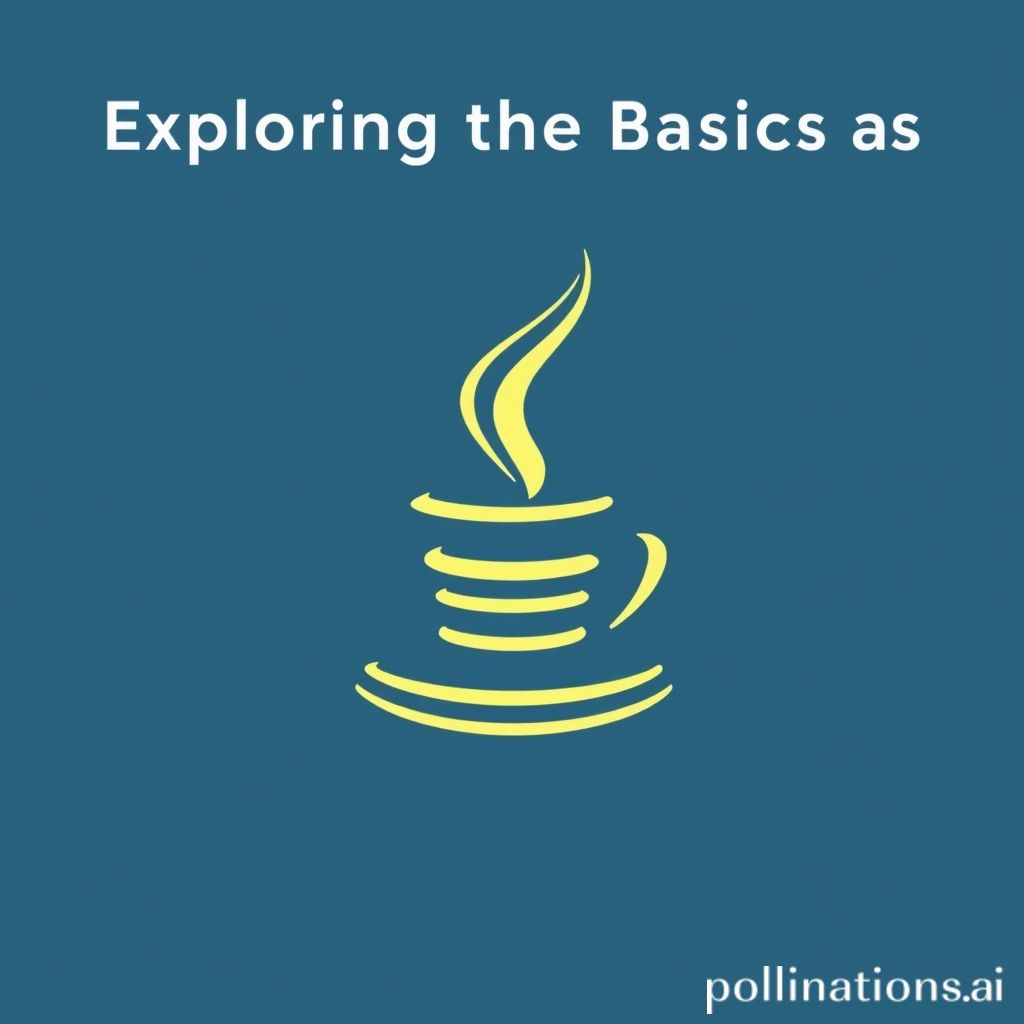
Exploring the Basics of Functional Programming in Java
Functional programming is a programming paradigm that treats computation as the evaluation of mathematical functions and avoids changing state and mutable data. Java, traditionally an object-oriented programming language, incorporated functional programming features starting from Java 8. This article delves into the basics of functional programming in Java.
Key Concepts of Functional Programming
Before diving into Java's implementation, it's essential to understand some core concepts of functional programming:
- First-Class Functions: In functional programming, functions are treated as first-class citizens. This means they can be passed as arguments, returned from other functions, and assigned to variables.
- Higher-Order Functions: These functions accept other functions as parameters and/or return them as results. They enable powerful abstractions and can help in writing less redundancy code.
- Pure Functions: A pure function has two main properties: it always returns the same result for the same inputs and does not cause any side effects. This predictability makes code easier to test and maintain.
- Immutable Data: In functional programming, data is immutable. Once created, data structures cannot be changed. This helps in avoiding unintended side effects and maintaining a clean state throughout the application.
Functional Interfaces in Java
Java’s introduction of functional programming is primarily facilitated by functional interfaces. A functional interface is an interface that contains only one abstract method. Java 8 introduced several built-in functional interfaces such as:
- Runnable: Represents a task that can be run.
- Callable: Similar to Runnable but can return a result and throw a checked exception.
- Consumer: Represents an operation that takes a single input argument and returns no result.
- Supplier: Represents a supplier of results. It does not take any input and returns a result.
- Function: Represents a function that takes one argument and produces a result.
- Predicate: Represents a boolean-valued function of one argument.
Lambda Expressions
One of the most significant features brought by Java 8 for functional programming is lambda expressions. A lambda expression allows you to create an instance of a functional interface using a concise syntax. Here is a simple example:
Functionsquare = x -> x * x; System.out.println(square.apply(5)); // Output: 25
In this example, we create a function that takes an integer and returns its square.
Stream API
The Stream API is another important feature that provides a functional approach to processing collections of objects. With the Stream API, you can perform complex operations such as filtering, mapping, and reducing with ease. Here’s a quick example:
Listnames = Arrays.asList("Alice", "Bob", "Charlie"); names.stream() .filter(name -> name.startsWith("A")) .forEach(System.out::println); // Output: Alice
Conclusion
Understanding functional programming is essential for modern Java developers. With the introduction of functional interfaces, lambda expressions, and the Stream API, Java has embraced functional programming capabilities, allowing developers to write cleaner and more efficient code. By mastering these concepts, you can enhance your programming skills and leverage Java's powerful features.