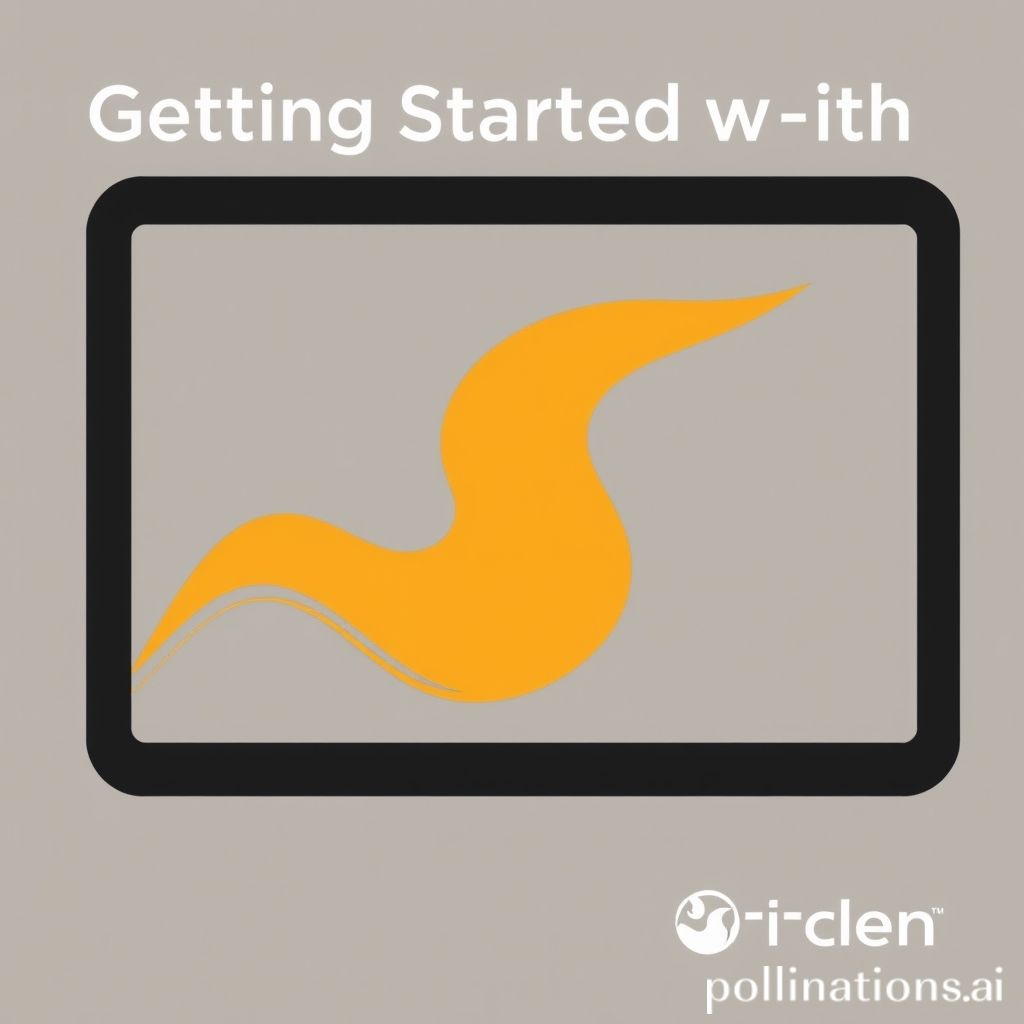
Getting Started with Swift: Developing iOS Applications
Swift is a powerful and intuitive programming language created by Apple for iOS, macOS, watchOS, and tvOS app development. If you're looking to dive into the world of iOS application development, understanding Swift is essential. This article will guide you through the basics of getting started with Swift and developing your first iOS application.
Setting Up Your Development Environment
Before you start coding in Swift, you'll need to set up your development environment. Here’s how:
- Install Xcode: Xcode is Apple's official integrated development environment (IDE) for macOS. It includes everything you need to build iOS applications, including a code editor, user interface designer, and testing tools.
- Get Familiar with Swift Playgrounds: Swift Playgrounds is an app for iPad and Mac that makes learning Swift interactive and fun. You can experiment with Swift code in a more relaxed environment.
- Join the Apple Developer Program: If you plan to distribute your app on the App Store, you’ll need to enroll in the Apple Developer Program, which provides access to app distribution tools, resources, and more.
Understanding Swift Basics
Once your environment is set up, the next step is to learn some Swift fundamentals:
- Variables and Constants: In Swift, you declare variables using the
var
keyword and constants usinglet
. Example:
var name = "John"
let age = 30
Int
, Double
, String
, and Bool
. Being aware of these types is crucial for writing efficient code.if
, switch
, and loops like for
and while
allow you to control how your code executes.Creating Your First iOS App
With a grasp of the basics, you’re ready to create your first iOS application:
- Open Xcode and Create a New Project: Select “Create a new Xcode project” from the welcome screen, choose “App” under the iOS tab, and click “Next”. Fill in your project name and other details.
- Design Your User Interface: Use Interface Builder to drag and drop elements like buttons, labels, and text fields onto your app’s main view.
- Write Your Code: In the ViewController.swift file, connect your user interface elements to your code using IBOutlets and IBActions. This will allow you to control your UI elements programmatically.
- Test and Debug: Use the iOS Simulator or a physical device to test your application. Debug any issues that arise using Xcode’s built-in debugging tools.
Further Resources
To continue your journey in iOS development with Swift, consider exploring the following resources:
By taking the time to learn Swift and explore iOS development, you'll be on your way to creating amazing applications that can reach millions of users. Happy coding!