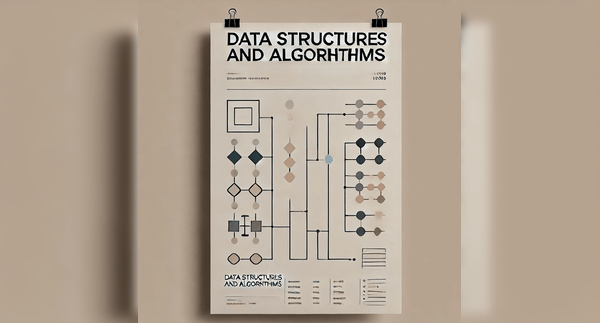
Itroduction
Data Structures and Algorithms (DSA) form the backbone of computer science and are crucial for developing efficient software. Whether you are a seasoned developer or a beginner, mastering DSA is essential for problem-solving, optimizing code, and acing technical interviews. In this guide, we will explore the fundamental concepts, types, and practical applications of Data Structures and Algorithms, providing you with the tools you need to excel in the field.
What are Data Structures?
Data structures are ways to store and organize data in a computer so that it can be accessed and modified efficiently. They are the building blocks of algorithms and software design. Common data structures include arrays, linked lists, stacks, queues, trees, and graphs, each offering unique advantages depending on the use case.
Types of Data Structures
- Arrays: A collection of elements stored in contiguous memory locations, ideal for quick access to elements but limited by a fixed size.
- Linked Lists: A sequence of elements where each element points to the next, offering dynamic size and ease of insertion or deletion.
- Stacks: A Last In, First Out (LIFO) data structure used in scenarios like function call management.
- Queues: A First In, First Out (FIFO) data structure, commonly used in scheduling and task management.
- Trees: Hierarchical structures with nodes connected by edges, used in databases and file systems.
- Graphs: Collections of nodes and edges, essential for representing networks like social media connections or transportation routes.
Understanding Algorithms
Algorithms are step-by-step procedures or formulas for solving problems. They operate on data structures to perform tasks such as searching, sorting, and processing data. Mastering algorithms allows programmers to write efficient and optimized code that performs well under various conditions.
Key Algorithm Concepts
- Time Complexity: A measure of the time an algorithm takes to complete as a function of the input size, often expressed using Big O notation.
- Space Complexity: A measure of the memory an algorithm uses relative to the input size.
- Recursion: A method where the solution to a problem depends on solutions to smaller instances of the same problem.
- Divide and Conquer: An approach that breaks a problem into smaller subproblems, solves each one independently, and combines the results.
- Greedy Algorithms: Algorithms that make the locally optimal choice at each step, aiming for a global optimum.
- Dynamic Programming: A method for solving complex problems by breaking them down into simpler subproblems and storing the results of subproblems to avoid redundant calculations.
The Importance of DSA in Programming
Understanding DSA is critical for writing efficient code that scales well with increasing data. It enables developers to choose the best data structure and algorithm for a given task, reducing runtime and memory usage. Additionally, DSA knowledge is a significant factor in technical interviews, where candidates are often tested on their ability to solve algorithmic problems efficiently.
Practical Applications of Data Structures and Algorithms
DSA is not just theoretical knowledge but has practical applications across various domains:
- Web Development: Efficient data structures like trees and hash tables are crucial for managing databases and ensuring quick data retrieval.
- Artificial Intelligence: Graph algorithms are widely used in AI for tasks like natural language processing and computer vision.
- Networking: Algorithms determine the best paths for data transmission across complex networks.
- Game Development: Trees and graphs are used to manage game states, AI decision-making, and rendering scenes efficiently.
Tips for Mastering Data Structures and Algorithms
To become proficient in DSA, consider the following tips:
- Practice Regularly: Consistent practice on platforms like LeetCode, HackerRank, and Codeforces helps in internalizing concepts.
- Understand the Basics: Ensure a strong grasp of fundamental data structures and algorithms before moving on to advanced topics.
- Analyze Solutions: After solving a problem, review other solutions to learn different approaches and optimize your code.
- Participate in Coding Competitions: Engaging in competitions hones problem-solving skills and helps in applying DSA knowledge under pressure.
- Study Interview Questions: Review common interview problems to prepare for technical interviews, focusing on efficient problem-solving techniques.
Conclusion
Mastering Data Structures and Algorithms is a vital step in becoming a proficient programmer. It not only enhances your problem-solving abilities but also makes you a more competitive candidate in the job market. By understanding the different types of data structures and algorithms, practicing regularly, and applying them to real-world problems, you can build a solid foundation in computer science that will serve you throughout your career.