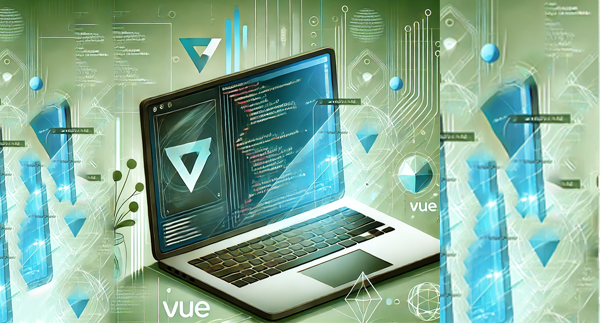
Intro
Vue.js is a popular and progressive JavaScript framework used for building user interfaces. It’s known for its simplicity and flexibility, making it a great choice for both beginners and experienced developers. In this article, we’ll walk you through the basics of Vue.js, including its core concepts, the creation of dynamic web applications, and best practices for using this powerful framework.
What is Vue.js?
Vue.js is an open-source JavaScript framework designed for building user interfaces and single-page applications. It was created by Evan You and has gained popularity due to its ease of use and integration capabilities with other projects and libraries.
Core Concepts of Vue.js
Understanding the core concepts of Vue.js is essential to mastering the framework. Here are some of the key features:
- Reactive Data Binding: Vue.js automatically updates the DOM whenever the state of the application changes, thanks to its reactivity system.
- Components: Vue.js applications are built using components, which are reusable and independent pieces of code that manage a part of the user interface.
- Directives: Directives are special tokens in the markup that tell the library to do something to a DOM element, like showing or hiding it.
- Vue CLI: The Vue Command Line Interface (CLI) is a powerful tool that allows developers to quickly scaffold projects and manage configurations.
Getting Started with Vue.js
To get started with Vue.js, you’ll first need to set up your development environment. This involves installing Node.js and NPM (Node Package Manager), and then using Vue CLI to create a new project:
npm install -g @vue/cli
vue create my-project
Once your project is set up, you can start building components, managing state, and integrating third-party libraries as needed.
Building Your First Vue.js Application
Let's build a simple application to display a list of items. Here’s a basic example:
<template>
<div>
<h1>Item List</h1>
<ul>
<li v-for="item in items" :key="item.id">{{ item.name }}</li>
</ul>
</div>
</template>
<script>
export default {
data() {
return {
items: [
{ id: 1, name: 'Item 1' },
{ id: 2, name: 'Item 2' },
{ id: 3, name: 'Item 3' }
]
}
}
}
</script>
This simple app dynamically renders a list of items stored in the component’s data. As you become more familiar with Vue.js, you can expand this basic example to include more features, such as user interaction, data fetching, and component communication.
Best Practices in Vue.js
When working with Vue.js, it’s important to follow best practices to ensure your code is maintainable and efficient. Here are some tips:
- Component Reusability: Break your application into small, reusable components.
- State Management: Use Vuex for managing state in larger applications.
- Code Organization: Keep your code modular and organized, following the separation of concerns principle.
- Performance Optimization: Leverage Vue's built-in tools to optimize performance, such as lazy loading and async components.
Conclusion
Vue.js is a powerful and flexible framework that can help you build dynamic and interactive web applications. By understanding its core concepts and following best practices, you can leverage its full potential. Start experimenting with Vue.js today, and you’ll soon see why it’s a favorite among developers worldwide.