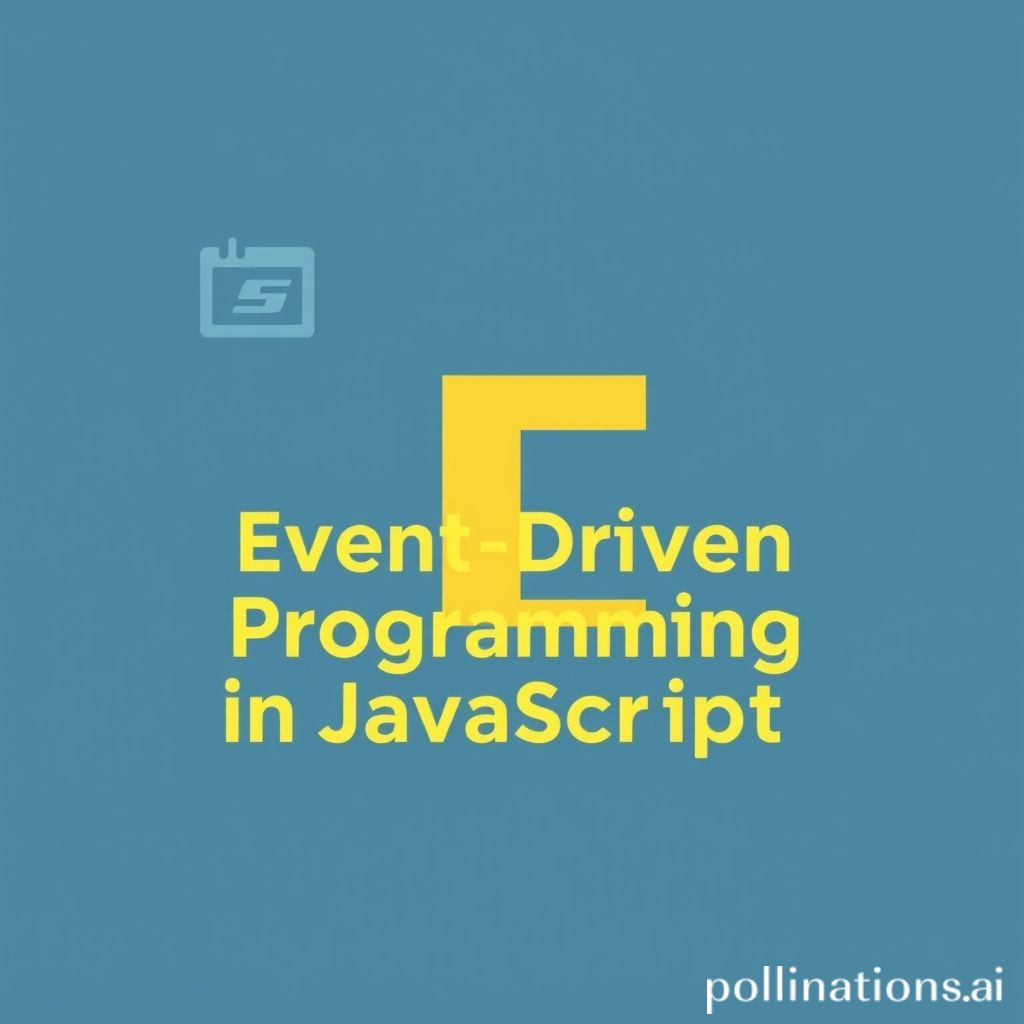
Understanding Event-Driven Programming in JavaScript
Event-driven programming is a programming paradigm that revolves around the concept of events, which are actions or occurrences that happen in the system. In JavaScript, this approach is particularly powerful due to its asynchronous nature and is extensively used for creating dynamic web applications.
What is an Event?
An event can be anything that happens in the browser environment that you can respond to with code. Common examples include:
- User interactions (clicks, keypresses)
- Page loading events
- Network requests (fetch responses)
- Timers (setTimeout, setInterval)
Events allow developers to create more interactive and responsive applications. Rather than executing code in a linear fashion, JavaScript can react to events as they occur, which helps in managing user interactions without blocking the main execution thread.
Event Handling in JavaScript
To work with events in JavaScript, developers utilize event listeners. An event listener is a function that waits for a particular event to occur, and when that happens, it executes a designated block of code. Adding an event listener can be done using the addEventListener
method.
Basic Syntax of addEventListener
The syntax for using addEventListener
is as follows:
element.addEventListener(event, handler);
Here, element
is the DOM element you want to listen to, event
is a string representing the event type (like 'click', 'keydown'), and handler
is the function that will run when the event occurs.
Example of Event-Driven Programming
Let’s look at a simple example of button click handling:
const button = document.getElementById('myButton');
button.addEventListener('click', function() {
alert('Button was clicked!');
});
In this example, we select a button element by its ID and attach a click event listener to it. When the button is clicked, an alert will display a message.
Advantages of Event-Driven Programming
- Asynchronous Processing: Enables non-blocking operations which improve the performance of web applications.
- Interactivity: Makes it easy to create responsive applications that provide a rich user experience.
- Modularity: Encourages separating concerns, which makes code more manageable and reusable.
Conclusion
Event-driven programming is fundamental to JavaScript, empowering developers to create interactive web applications. By leveraging events and listeners, developers can respond to user inputs, manage asynchronous operations, and ultimately enhance the overall user experience. Understanding how to implement and manage events will significantly improve your JavaScript programming skills.